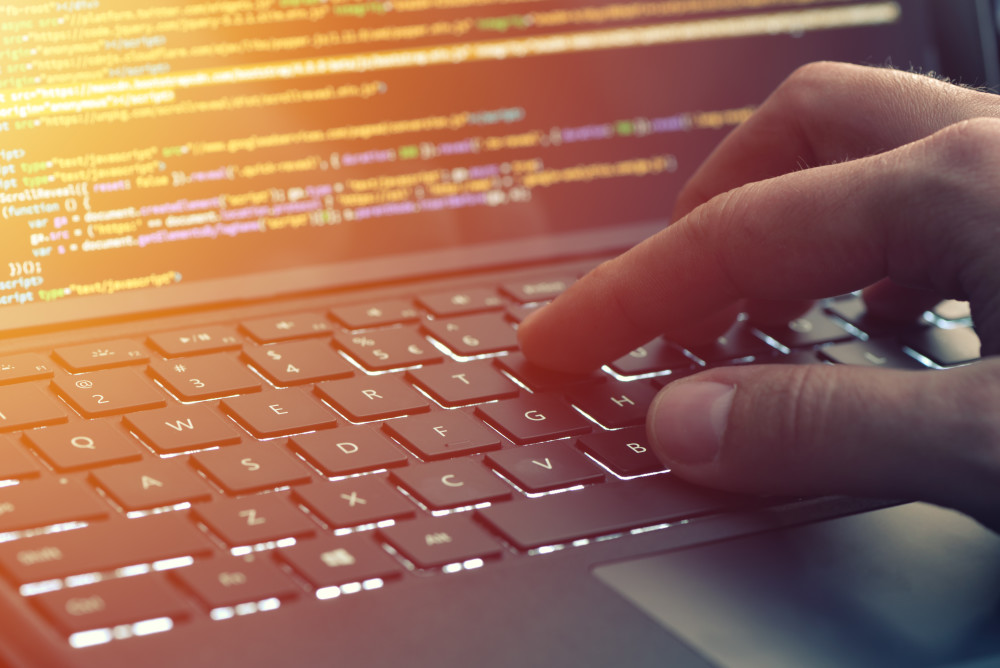
It's a very common task to work with an array of values, each of the same type. Integers, strings, all kinds of objects etc. But PHP is still a weakly typed language, so it's hard to tell if an arbitrary array actually contains only values of a given type.
Of course, you can always use a class:
```
class IntArray {
private $values = [];
public function add(int $value) {
$this->values[] = $value;
}
}
```
Then, whenever you need an array of integers, you may write something like this:
```
class BatchProcessor
{
private $ids;
public function __construct(IntArray $ids) {
$this->ids = $ids;
}
}
```
Not bad. You'll need a class per type, though, and that may seem a bi...
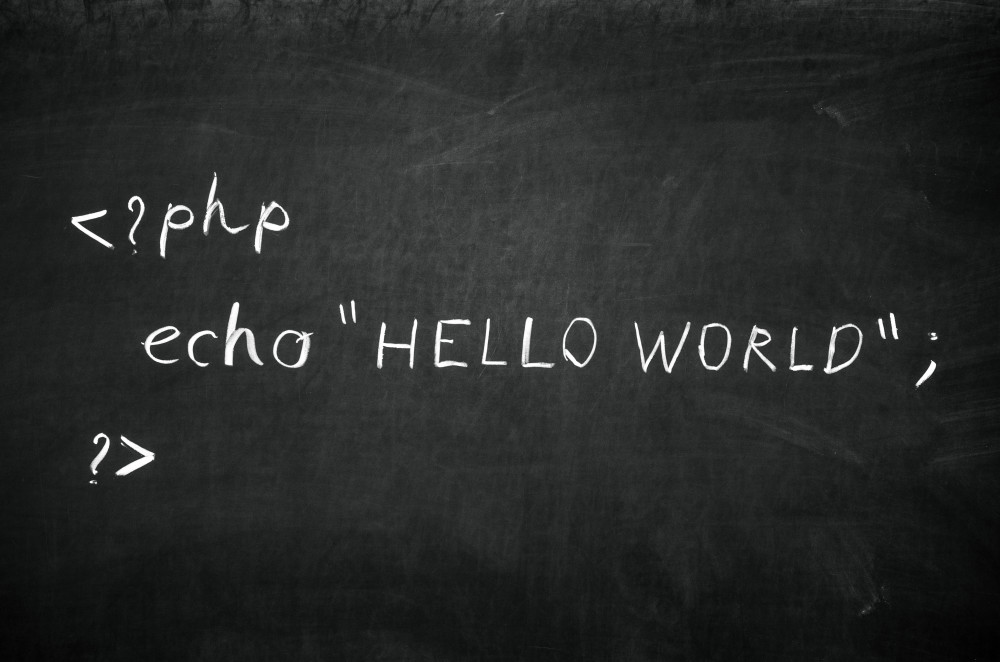
It's 2018, and you're a top-notch modern web developer, with a load of knowledge and tools right there at your disposal: Google and StackOverflow, debugger with a GUI, IDE with autocomplete, you name it. Occasionally, though, you still find yourself in a plain old text console on a remote server, or you have to do something without IDE, or there is no network connection... In such cases it might be helpful to feel comfortable in a simple terminal. In this post I'm going to list some switches for the PHP command that you can use to get information and some utilities.
### Getting basic information about PHP
```
$ php -i
phpinfo()
PHP Version => 7.2.10-0ubuntu1
System => Linux awesome 4.18.0-10-generic #11-Ubuntu SMP Thu Oct 11 15:13:55 UTC 2018 x86_64
Build Date...
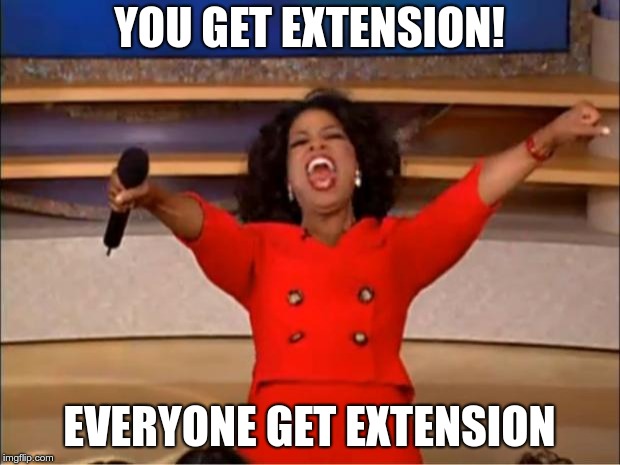
Ever wanted to publish your own extension for PHP but stopped by the lack of C language background? Well, maybe it's time for another take. [Zephir language](https://zephir-lang.com/en) is targeted at people like you.
If you follow this link, you will find these words that say a lot about this project:
> Zephir, an open source, high-level language designed to ease the creation and
maintainability of extensions for PHP with a focus on type and memory safety.
Its syntax highly resembles that of PHP, only there's no dollars scattered around your code. Dollar signs, I mean, the PHP `$variables`. You only can create object oriented extensions, and all the classes written in Zephir must be namespaced. A different and stricter type system exists in Zephir, which allows for transpiling the code you write, into a real C extension.
Why...